学习JavaMinIO本地部署MinIO通过Java实现文件上传
Onew添加依赖
打开pom.xml文件,将的 MinIO依赖添加进去,并保存文件,示例代码为:
1 2 3 4 5
| <dependency> <groupId>io.minio</groupId> <artifactId>minio</artifactId> <version>8.5.10</version> </dependency>
|
新建Maven项目
右键单击文件编辑区域,选择Maven>>>Reload Project,或在右侧的Maven工具窗口中点击刷新按钮。
新建Maven项目
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60
| package org.example;
import io.minio.MinioClient; import io.minio.errors.MinioException; import io.minio.BucketExistsArgs; import io.minio.MakeBucketArgs; import io.minio.PutObjectArgs;
import java.io.FileInputStream; import java.io.IOException; import java.io.InputStream; import java.security.InvalidKeyException; import java.security.NoSuchAlgorithmException;
public class MinioUploader {
public static void main(String[] args) { // MinIO 服务器信息 String endpoint = "http://localhost:9000"; String accessKey = "YOUR_ACCESS_KEY"; String secretKey = "YOUR_SECRET_KEY"; String bucketName = "YOUR_BUCKET_NAME";
// 要上传的文件信息 String objectName = "example.jpg"; // 文件在 MinIO 中的对象键 String filePath = "path/to/your/file/example.jpg"; // 本地文件路径
// 初始化 MinIO 客户端 try { MinioClient minioClient = MinioClient.builder() .endpoint(endpoint) .credentials(accessKey, secretKey) .build();
// 检查存储桶是否存在,不存在则创建 boolean isExist = minioClient.bucketExists( BucketExistsArgs.builder().bucket(bucketName).build()); if (!isExist) { minioClient.makeBucket( MakeBucketArgs.builder().bucket(bucketName).build()); }
// 使用文件流上传文件 try (InputStream inputStream = new FileInputStream(filePath)) { minioClient.putObject( PutObjectArgs.builder() .bucket(bucketName) .object(objectName) .stream(inputStream, inputStream.available(), -1) .build()); System.out.println("File uploaded successfully."); } catch (IOException e) { e.printStackTrace(); }
} catch (MinioException | NoSuchAlgorithmException | InvalidKeyException | IOException e) { e.printStackTrace(); } } }
|
上传结果
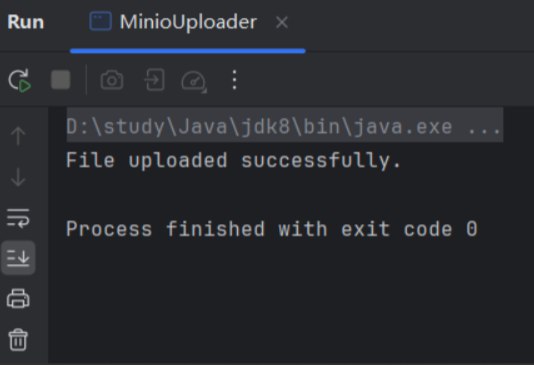